How does .reduce() work?
Reduce method executes a reducer function on each element of the array.
Okay, but what is this reducer function?
It is a function that takes accumulator (first parameter) and current value (second parameter) from an iteration, do the calculations, then in the end the accumulator is returned as a result of the reduce function.
Let’s look on the example:
We have got the wishlist array with objects inside. Our goal is to sum every item’s price.
const wishlist = [
{ title: "Tesla Model 3", price: 90000 },
{ title: "Diamond ring", price: 5000 },
{ title: "Snack", price: 5 },
{ title: "Gold watch", price: 40000 },
{ title: "Bigger gold watch", price: 90000 },
];
const shoppingSpree = (arr) => arr.reduce((acc, el) => acc + el.price, 0);
console.log(shoppingSpree(wishlist)); // 225005
As you can see from the console.log, the sum is correct and reduce function works fine.
Now let’s console log the accumulator (acc) inside our function.
The output from each iteration of the loop is below:

During the first iteration, the acc equals to 0, which is correct, then we are adding Tesla’s price to it, so after the next iteration acc equals to tesla’s price, then we are adding the diamond ring’s price etc.
In the end the reducer function returns acc as the final result.
Syntax:
According to the MDN:
// Arrow function
reduce((previousValue, currentValue, currentIndex, array) => { /* … */ }, initialValue)
// Callback function
reduce(callbackFn, initialValue)
// Inline callback function
reduce(function(previousValue, currentValue, currentIndex, array) { /* … */ }, initialValue)
Callback function
The callback is a reducer function and it takes the accumulator (previousValue) and current value (currentValue) from an iteration. In addition, we can also use the current index of the element (currentIndex) and current array (array).
accumulator
This value represents some kind of ‘work in progress’ of the returned value from the function.
currentValue
The current element in the array.
currentIndex
The index of the current element.
currentArray
The source array.
initialValue
This is completely optional value. If it is given, and the array has any element in it, the first call to callback function will receive this value as its accumulator.
Understanding how reduce() work by diagram
Here is the Ashutosh Biswas's diagram about how reduce and reduceRight works:
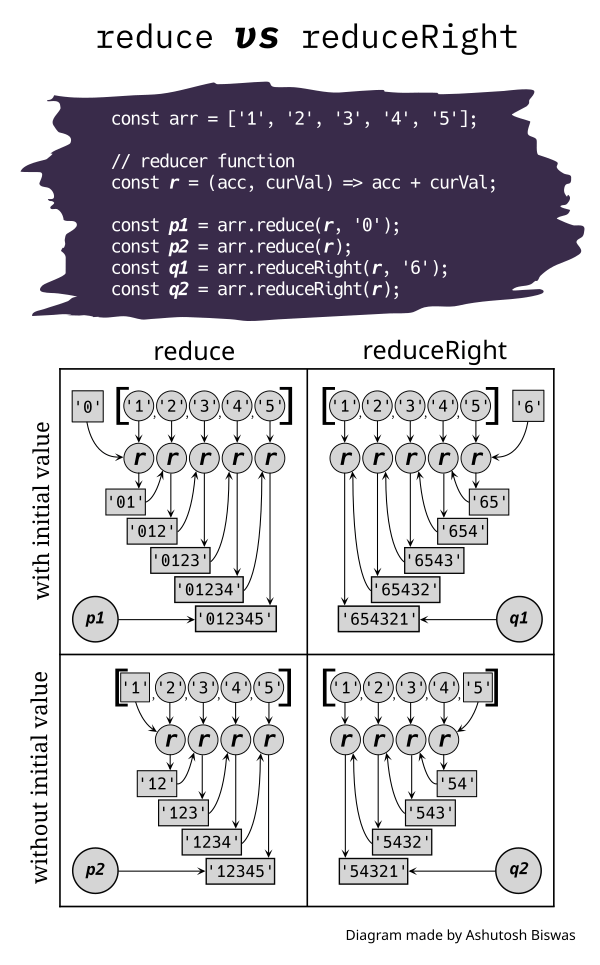
Additional informations
It’s worth noting that we can also use reduceRight() method, which does the same stuff but in opposite direction. It just starts from end end of the array to the beggining.