Undoubtedly, one of the most frequently used array methods is the .map() method.
How does this method work? Let's use the following notation:
list.map(function callback(element, index, array) {
// body
});
As MDN says:
.map() method calls a provided callback function once for each element in an array, in order, and constructs a new array from the results.
Callback function is invoked only for array indexes which have assigned values. It is not invoked for empty slots in sparse arrays.
Callback function is invoked with three arguments
- The value of the element
- The index of the element
- The array object being mapped.
The .map() function iterates through the array and returns a new array element each time. When the loop ends, a new array that contains the same number of elements is returned.
const numbers = [1, 2, 3];
const newArray = numbers.map(el => el * 2);
console.log(newArray); // [2, 4, 6]
Let's now use a slightly more detailed example. Here we have an array named 'people' containing objects. Each of them contains a person's name, age and profession:
const people = [
{
name: "John",
age: 28,
profession: "Medical assistant"
},
{
name: "Mark",
age: 39,
profession: "Bartender"
},
{
name: "Rachel",
age: 32,
profession: "Mechanic"
},
{
name: "Nate",
age: 47,
profession: "Truck driver"
},
{
name: "Jeniffer",
age: 45,
profession: "Lawyer"
},
];
Let's create a new array that returns sentences describing a person's name, age and profession.
We will only use the first parameter of the callback function, which is the current element of the array we are iterating over.
const list = people.map((person) => {
return `${person.name} is ${person.age} years old and works as a ${person.profession}`;
});
Now let's display our list in the console and see how it looks like.
console.log(list);
This is the output:
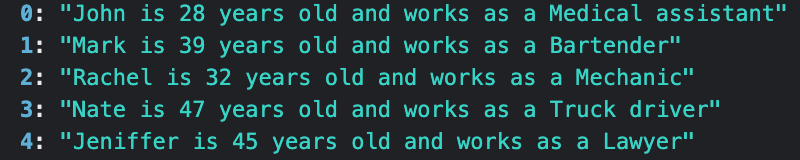
As you can see, everything looks as we assumed at the beginning.
Now let's try to use the second parameter of the callback function - the index of the current element and display person's index.
const list = people.map((person, index) => {
return `${person.name} is ${person.age} years old and works as a ${person.profession} and also is located in the array as index number ${index}`;
});
Again, we are displaying a variable named 'list' in the console and getting this output:

As you can see, everything looks as we assumed at the beginning.
To improve readability a bit, we can use destructuring of course.
const list = people.map(({ name, age, profession }) => {
return `${name} is ${age} years old and works as a ${profession}`;
});
The effect will be the same and our code looks more concise and readable.
In addition, we can get rid of the curly braces, the return keyword and write all the code in one line:
const list = people.map(({ name, age, profession }) =>`${name} is ${age} years old and works as a ${profession}`);
Actually, all of the above ways are correct, and it is hard to determine which is the best. It is a rather subjective perception. Choosing one of them is a matter of agreement between developers working on the same project.