How does .find() method work?
The find() method returns the first element from the array that matches the provided function.
If there are no values that matches the function, undefined is returned.
Quick example:
We are looking for first object, which value is bigger than 100k and less than 280k
const shop1 = {
owner: "Carl Johansson",
value: "495000",
};
const shop2 = {
owner: "Thomas Davis",
value: "250000",
};
const shop3 = {
owner: "Steven Jackson",
value: "100000",
};
const shops = [shop1, shop2, shop3];
const shop = shops.find((shop) => shop.value > 100000 && shop.value < 280000);
console.log(shop);
The output:
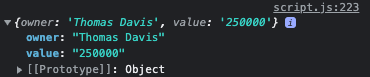
Syntax
According to the MDN:
// Arrow function
find((element, index, array) => { /* … */ } )
// Inline callback function
find(function(element, index, array) { /* … */ }, thisArg)
// Callback function
find(callbackFn, thisArg)
Callback function
Function that is executed on each element in the array.
The callback function is called with three arguments:
- element - the current element in the array
- index - index of the current element
- array - current array
thisArg
Object to use as this inside callback function. If it’s not provided, the undefined is used.
thisArg is an optional argument.
Details
The find method executes the callback function once for each element from the array until the callback function return a truthy value.
The find method does not mutate the array on which it is called itself.
The find method is generic.
What about findIndex()?
The key difference between find() and findIndex() methods is that findIndex() returns the index (not the element itself) of the first element in the array that matches the provided testing function.
If no elements pass the test function, -1 is returned.
The syntax looks the same as ind find() method.
The findIndex() method is also generic.
According to the shops array from first example:
const shop = shops.findIndex(
(shop) => shop.value > 100000 && shop.value < 280000
);
console.log(shop);
The output:
