How does .filter() work?
The filter() method creates a new array with only elements that pass the test defined by the given function. Elements which do not pass the test are skipped and are not included in the new created array.
const filterEvenLengthWords = (words) => {
const result = words.filter((word) => word.length % 2 === 0);
console.log(result);
};
filterEvenLengthWords(["Hello", "People"]);
Output:

Another example:
const numbers = [1, 3, 5, 7, 8, 9, 10, 11];
const greaterThanFive = (input) => input.filter((item) => item > 7);
console.log(greaterThanFive(numbers));
Output:
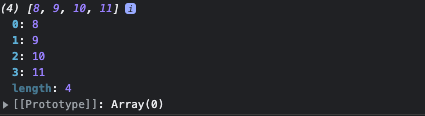
Syntax:
According to the MDN:
// Arrow function
filter((element, index, array) => { /* … */ } )
// Callback function
filter(callbackFn, thisArg)
// Inline callback function
filter(function(element, index, array) { /* … */ }, thisArg)
Callback function
The callback function tests each element of the array and returns true if element passed the test or false if it does not.
The function is called with three arguments:
- element - The current element in the array
- index - The index of the current element in the array
- array - Current array
thisArg
Except the Callback function, we have access to the second parameter - thisArg.
thisArg is optional and represents value to use as this keyword when executing callback function.
Additional informations
The filter() method same as any of array methods is generic.
This means that filter() method access the array elements through the length property and the indexed elements but It does not access any internal data of the array object.
According to that filter() method can be called too on the array-like objects.
The filter() method is copying method. It means that it does not mutate the existing array but instead returns a shallow copy array with some filtered out elements.